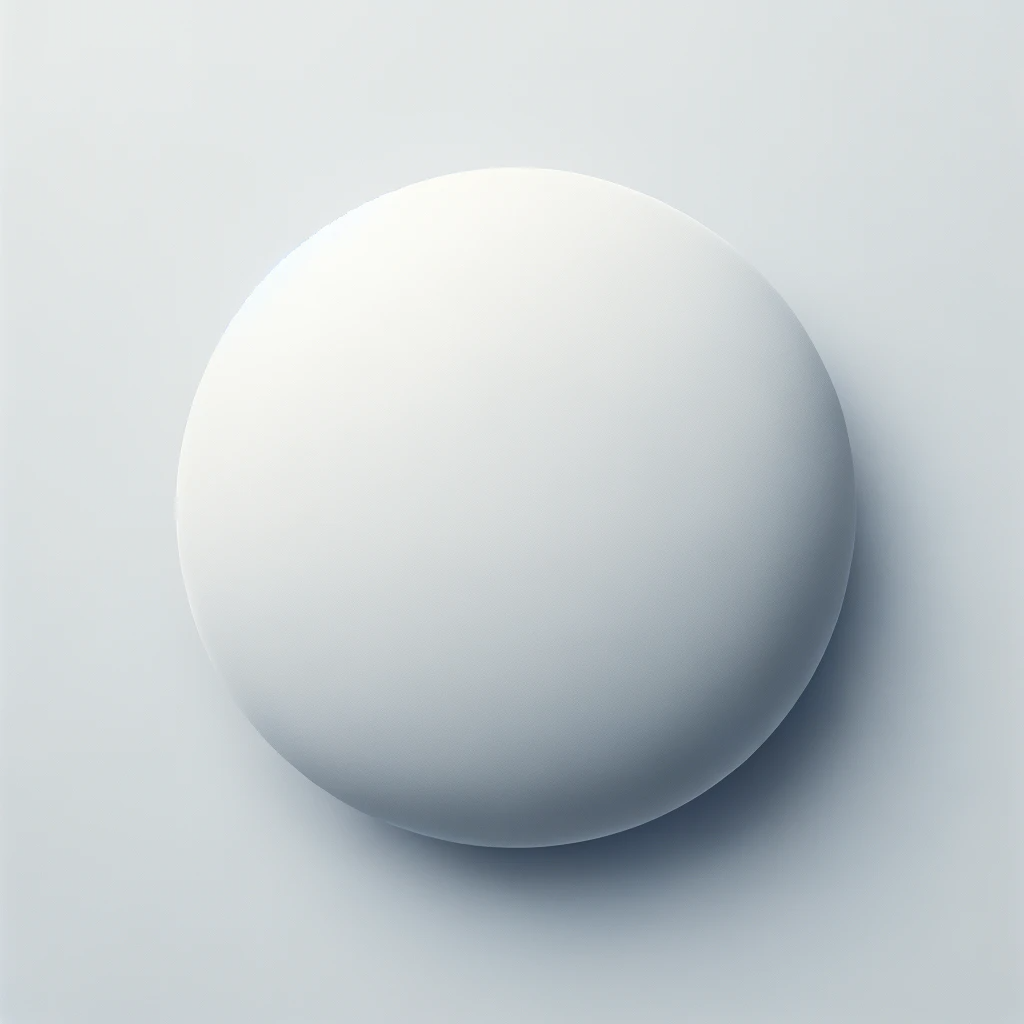
Viewed 12k times. 3. When I was a python beginner, I could create a multiple lines for loop that make a list of 1~100: a=[] for i in range(1,101): a.append(i) When I knew how to write a single line for loop, I could simply my code. a=[ _ for _ in range(1,101)]Evaluate an expression node or a string containing only a Python literal or container display. The string or node provided may only consist of the following Python literal structures: strings, bytes, numbers, tuples, lists, dicts, sets, booleans, None and Ellipsis.A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example.Here is how you can test which piece of code is faster: % python -mtimeit "l=[]" 10000000 loops, best of 3: 0.0711 usec per loop. % python -mtimeit "l=list()" 1000000 loops, best of 3: 0.297 usec per loop. However, in practice, this initialization is most likely an extremely small part of your program, so worrying about this is probably wrong ...One way to create a list of lists in Python is by using the append () method within a loop. You can initialize an empty list and then use append () to add individual lists as elements to the outer list. list_of_lists = [] # Append individual lists to the outer list. list_of_lists.append([1, 2, 3])5. This implementation does the same as yours for "square" lists of lists: def makeLRGrid(g): return [row[:] for row in g] A list can be copied by slicing the whole list with [:], and you can use a list comprehension to do this for every row. Edit: You seem to be actually aiming at transposing the list of lists.Python then finds itself stuck in a loop where neither import can complete first. Since app hasn't finished initializing (it's waiting to complete the import before …How to Create An Empty Dictionary in Python. To create an empty dictionary, first create a variable name which will be the name of the dictionary. Then, assign the variable to an empty set of curly braces, {}. Another way of creating an empty dictionary is to use the dict () function without passing any arguments.A fixed-size list is a list that has a predefined number of elements and does not change in size. In Python, while lists are inherently dynamic, we can simulate ...In today’s fast-paced world, staying organized and managing your time effectively is crucial. One powerful tool that can help you achieve this is Google Calendar. Not only can you ...According to the Smithsonian National Zoological Park, the Burmese python is the sixth largest snake in the world, and it can weigh as much as 100 pounds. The python can grow as mu...To create a stack in Python you can use a class with a single attribute of type list. The elements of the stack are stored in the list using the push method and are retrieved using the pop method. Additional methods allow to get the size of the stack and the value of the element at the top of the stack.Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ...If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using …Create a List with List function in Python. Create a List with range function in Python. Create a List with append method in Python. Create a List …Viewed 12k times. 3. When I was a python beginner, I could create a multiple lines for loop that make a list of 1~100: a=[] for i in range(1,101): a.append(i) When I knew how to write a single line for loop, I could simply my code. a=[ _ for _ in range(1,101)]Dey 9, 1402 AP ... Make the API return a python list or something I can rely will be a python list · you are provided a list of entities to discover within a text; ...SHALLOW COPY: new_object = copy.copy(original_object) DEEP COPY: new_object = copy.deepcopy(original_object) With a shallow copy a new compound object is created (e.g. a list of lists) and references to the objects found in the original object are added to the new compound object.How to Create a List in Python. To create a list in Python, we use square brackets ( [] ). Here's what a list looks like: ListName = [ListItem, …Classification: Python is a high-level object-oriented scripting language. Usage: Back-end web developers use Python to create web …Pseudo-Code. def main(): create an empty list value = getInput() while value isnt zero: add value to the list value = getInput() printOutput(list) def getInput(): prompt the user for a value make sure that the value is an int (convert to int) return the number def printOutput(list): print out the number of input values print out the individual input values …I want to create a series of lists with unique names inside a for-loop and use the index to create the liste names. Here is what I want to do x = [100,2,300,4,75] for i in x: list_i=[] I want...Having a baby is an exciting time, and one of the many things expectant parents need to think about is creating a baby gift registry list. This helps family and friends know what i...Feb 2, 2012 · I want to create a list of dates, starting with today, and going back an arbitrary number of days, say, in my example 100 days. Is there a better way to do it than this? import datetime a = datetime.datetime.today() numdays = 100 dateList = [] for x in range (0, numdays): dateList.append(a - datetime.timedelta(days = x)) print dateList Sep 25, 2022 · list2 = list (dict) print (list2) [‘name’, ‘age’, ‘gender’] Converting a dictionary using list (dict) will extract all its keys and create a list. That is why we have the output [‘name’,’age’,’gender’] above. If we want to create a list of a dictionary’s values instead, we’ll have to access the values with dict ... 1 Answer. Sorted by: 3. The pythonic way to do this is: ["abc"]*6. As jonsharpe commented, if you elements are mutable, this will create elements with the same reference. To create a new object in each case, you could do. [[] for _ in range(6)] Share.Mordad 23, 1402 AP ... In Python, there are two primary methods to create an empty list: using square brackets [] and using the list() function. This section will ...Creating list from list in python based on an if condition. 0. Making new list of list with some condition of other list. 1. Create a new list from another list based on condition. 0. If condition in Python and create list. 2. Conditionally create list of lists. 1. Creating lists based on conditional value. 2.Feb 12, 2024 · This result is obtained by calling the create_number_list function with an argument of 12. The function generates a list containing numbers from 1 to 12 (inclusive), and the printed output shows the resulting list. How To Create a List of Numbers from 1 to N in Python Using the range() Function @loved.by.Jesus: Yeah, they added optimizations for Python level method calls in 3.7 that were extended to C extension method calls in 3.8 by PEP 590 that remove the overhead of creating a bound method each time you call a method, so the cost to call alist.copy() is now a dict lookup on the list type, then a relatively cheap no-arg function call that ultimately …To create a list from this iterator, we can use the list function. The time complexity of the itertools.repeat approach is O(n), where n is the number of elements in the list. This is because the list function needs to iterate over the entire iterator produced by itertools.repeat in order to create the final list.In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append: my_list = [] for i in range(50): …Be aware, that in Python 3.x map() does not return a list (so you would need to do something like this: new_list = list(map(my_func, old_list))). Filling other list using simple for ... in loop. Alternatively you could use simple loop - it is still valid and Pythonic: new_list = [] for item in old_list: new_list.append(item * 10) GeneratorsIn Python, a list is a sequence data type. It is an ordered collection of items. Each item in a list has a unique position index, starting from 0. A list in Python is similar to an array in C, C++ or Java. However, the major difference is that in C/C++/Java, the array elements must be of same type. On the other hand, Python lists may have ...Shahrivar 24, 1401 AP ... Trying to create a list of even numbers from a list but receiving an error. Here's what I have: def myfunc(*args): myfunc=[2,3,4,6 ...5 Answers. The normal, regular way to handle this sort of requirement is to keep the keyed lists in a dictionary, not as variable names with a suffix. my_list[i] = [] for j in range(10): my_list[i].append(j) What you are doing here is that, you are trying to assign a list to a string. You cannot do that.The syntax for the “not equal” operator is != in the Python programming language. This operator is most often used in the test condition of an “if” or “while” statement. The test c...Note that in the third case, since lists are referred to by reference, changing one instance of ['x','y'] in the list will change all of them, since they all refer to the same list. To avoid referencing the same item, you can use a comprehension instead to create new objects for each list element: L = [['x','y'] for i in range(20)]Jan 30, 2024 · Here, I will explain different examples of how to use square brackets to create a list in Python, such as creating a list with an empty list, with different elements of different data types, etc. Below, you can see the code that I have mentioned to create a list in Python using different conditions. If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using …This way, we can Convert the list to set in Python using set().. Method 2: Python convert list into set using set comprehension. Set comprehension provides a concise way to create sets in Python, similar to how list comprehension works for lists. It’s useful when needing to filter or apply a transformation to Python list elements during …How many more reports can you generate? How many sales figures do you have to tally, how many charts, how many databases, how many sql queries, how many 'design' pattern to follow...Since append mutates the list, this is a bit difficult to express recursively. One way you could do this is by using a separate inner function that passes on the current L to the next recursive call. def rec(S): def go(S, L): if len(S) > 0: L.append(S[-1]) return go(S[0:-1], L) else: return L.According to the Python Documentation: If no argument is given, the constructor creates a new empty list, []. 💡 Tip: This creates a new list object in memory and since we didn't pass any arguments to list (), an empty list will be created. For example: num = list () This empty list will have length 0, as you can see right here:I would like to set a list of hosts (IPs) on which my Flask server can listen on so that if the main Ethernet port blows up there is the other one that can …In this example, we have a list my_list with five elements. We want to remove the element with index 2, which has the value 3. We use the del statement and specify the index of the item we want to remove. After removing the element, we print the updated list.The first one is a generator expression and the second one is a list comprehension. You can found some informations here: Official Tutorial on List Comprehension, PEP 289. And here in some OS questions: Generator Expressions vs. List Comprehension, generator-comprehension. –Sep 25, 2022 · list2 = list (dict) print (list2) [‘name’, ‘age’, ‘gender’] Converting a dictionary using list (dict) will extract all its keys and create a list. That is why we have the output [‘name’,’age’,’gender’] above. If we want to create a list of a dictionary’s values instead, we’ll have to access the values with dict ... Create Lists. Python Basics: Lists and Tuples Martin Breuss 06:33. Mark as Completed. Supporting Material. Transcript. Discussion. 00:00 In this lesson, …Python then finds itself stuck in a loop where neither import can complete first. Since app hasn't finished initializing (it's waiting to complete the import before …Take the thing l [0], e.g. "A", from the line and assign the numeric value int (), e.g. 1, of the measurement in the respective column l [col], e.g. "1", to the thing. Then use a dictionary comprehension to combine it into the next line of the desired result. Finally append () the dict to the result list ld.Set. Sets are used to store multiple items in a single variable. Set is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Tuple, and Dictionary, all with different qualities and usage. A set is a collection which is unordered, unchangeable*, and unindexed. * Note: Set items are unchangeable, but you ... If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using np.random.randint is the fastest option. In Python, creating a list of lists using a for loop involves iterating over a range or an existing iterable and appending lists to the main list. This approach allows for the dynamic generation of nested lists based on a specific pattern or condition, providing flexibility in list construction. In this article, we will …Python append() to Clone or Copy a list. This can be used for appending and adding elements to list or copying them to a new list. It is used to add elements to the last position of the list. This takes around 0.325 seconds to complete and is the slowest method of cloning. In this example, we are using Python append to copy a Python list.Some python adaptations include a high metabolism, the enlargement of organs during feeding and heat sensitive organs. It’s these heat sensitive organs that allow pythons to identi... for loop in Python creating a list. 1. Create list from for loop. 0. Creating a list through looping. 4. Generating pairs from python list. 0. Creating pairs of ... Python is a versatile programming language that is widely used for game development. One of the most popular games created using Python is the classic Snake Game. To achieve optima...To create a list from this iterator, we can use the list function. The time complexity of the itertools.repeat approach is O(n), where n is the number of elements in the list. This is because the list function needs to iterate over the entire iterator produced by itertools.repeat in order to create the final list.Ordibehesht 14, 1399 AP ... This Video will help you to understand how to create a list in python • What is List? • How to use List • Assigning multiple values to List ...NumPy is the fundamental Python library for numerical computing. Its most important type is an array type called ndarray.NumPy offers a lot of array creation routines …To create a stack in Python you can use a class with a single attribute of type list. The elements of the stack are stored in the list using the push method and are retrieved using the pop method. Additional methods allow to get the size of the stack and the value of the element at the top of the stack.5. This implementation does the same as yours for "square" lists of lists: def makeLRGrid(g): return [row[:] for row in g] A list can be copied by slicing the whole list with [:], and you can use a list comprehension to do this for every row. Edit: You seem to be actually aiming at transposing the list of lists.Create an empty list with certain size in Python (18 answers) Multidimensional array in Python (12 answers) Closed 7 months ago . Be aware, that in Python 3.x map() does not return a list (so you would need to do something like this: new_list = list(map(my_func, old_list))). Filling other list using simple for ... in loop. Alternatively you could use simple loop - it is still valid and Pythonic: new_list = [] for item in old_list: new_list.append(item * 10) Generators . here if the file does not exist with the mentionedIf we compare the runtimes, among random list generators, random Do you find yourself spending too much time manually entering data into your Excel spreadsheets? Are you tired of dealing with typos and inconsistencies? Creating a dynamic drop-do...as it creates a list of references to the same empty dictionary, so that if you update one dictionary in the list, all the other references get updated too. Try these updates to see the difference: dictlistGOOD[0]["key"] = "value". dictlistFAIL[0]["key"] = "value". (I was actually looking for user1850980's answer to the question asked, so his ... My +1 for "construct" as it is consi I would like to set a list of hosts (IPs) on which my Flask server can listen on so that if the main Ethernet port blows up there is the other one that can …1 Answer. Sorted by: 3. The pythonic way to do this is: ["abc"]*6. As jonsharpe commented, if you elements are mutable, this will create elements with the same reference. To create a new object in each case, you could do. [[] for _ in range(6)] Share. In Python, “strip” is a method that eliminates specific characte...
Continue Reading