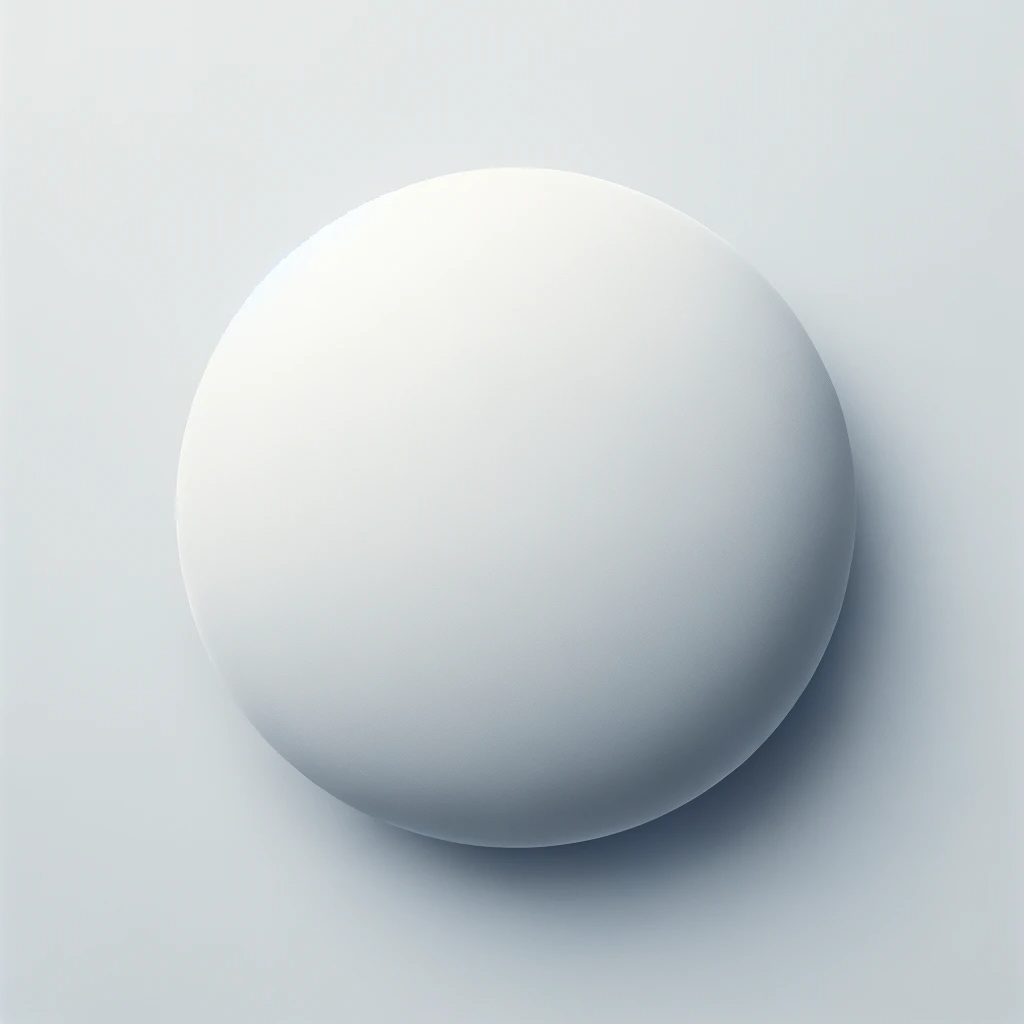
@Steve already gave a good answer to your question: verts = [None] * 1000 Warning: As @Joachim Wuttke pointed out, the list must be initialized with an immutable element.[[]] * 1000 does not work as expected because you will get a list of 1000 identical lists (similar to a list of 1000 points to the same list in C). Immutable objects like int, str …But I realized that most examples make use of the column variable matching a key in the dict and then assigning the value to the column, but in my case I want to …Everything in Python is an object, including lists. All objects have a header of some sort in the C implementation. Lists and other similar builtin objects with a "size" in Python, in particular, have an attribute called ob_size, where the number of elements in the object is cached.Python Lists (With Examples) List can be seen as a collection: they can hold many variables. List resemble physical lists, they can contain a number of items. A list can have any number of elements. They are similar to arrays in other programming languages. Lists can hold all kinds of variables: integers (whole numbers), floats, characters ...Preallocate Storage for Lists. The first and fastest way to use the * operator, which repeats a list a specified. number of times. >>> [None] * 10 [None, None, None, None, None, None, None, None, None, None] A million iterations (default value of iterations in timeit) take approximately. 117 ms.( thanx Robert for clarification, below is only in case of using listcomprehensions : )! another very important difference is that with round brackets we will have a generator and so the memory consumption is much lower in comparison to list with square brackets esspecially when you deal with big lists - generator will eat not only significantly less memory but …Feb 2, 2012 · I want to create a list of dates, starting with today, and going back an arbitrary number of days, say, in my example 100 days. Is there a better way to do it than this? import datetime a = datetime.datetime.today() numdays = 100 dateList = [] for x in range (0, numdays): dateList.append(a - datetime.timedelta(days = x)) print dateList A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.python List from function. 1. List as Function Arguments in Python. 0. Use list as function definition parameters in python. 0. Using a list as parameters for a function. Hot Network Questions Questions about how to proceed when residuals of linear regression are not exactly normally distributedPython has become one of the most widely used programming languages in the world, and for good reason. It is versatile, easy to learn, and has a vast array of libraries and framewo...How to Create a List in Python. You can create a list in Python by separating the elements with commas and using square brackets []. Let's create an … W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Classes — Python 3.12.2 documentation. 9. Classes ¶. Classes provide a means of bundling data and functionality together. Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state. Class instances can also have methods ...Mar 1, 2024 · In this approach we use the * operator with a list containing the default value to create a list with the specified size, where each element is a reference to the same object. Python3. size = 5. placeholder_value = 0. my_list = [placeholder_value] * size. print(my_list) Output. [0, 0, 0, 0, 0] @Steve already gave a good answer to your question: verts = [None] * 1000 Warning: As @Joachim Wuttke pointed out, the list must be initialized with an immutable element.[[]] * 1000 does not work as expected because you will get a list of 1000 identical lists (similar to a list of 1000 points to the same list in C). Immutable objects like int, str …However, lists are quite slow for this purpose because inserting or deleting an element at the beginning requires shifting all of the other elements by one, requiring O(n) time. The code simulates a queue using a Python list. It adds elements ‘a’, ‘b’, and ‘c’ to the queue and then dequeues them, resulting in an empty queue at the end.In Python, “strip” is a method that eliminates specific characters from the beginning and the end of a string. By default, it removes any white space characters, such as spaces, ta...list consists of RANDOM strings inside it #example list = [1,2,3,4] filename = ('output.txt ... 'w') outfile.writelines([str(i)+'\n' for i in some_list]) outfile.close() In Python file objects are context managers which means they can be used with a with statement so you could do the same thing a little more succinctly with the ...Dec 30, 2023 ... Make the API return a python list or something I can rely will be a python list · you are provided a list of entities to discover within a text; ...Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python.Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ...You can sort a list in Python using the sort () method. The sort () method allows you to order items in a list. Here's the syntax: list.sort(reverse=True|False, key=sortFunction) The method accepts two optional arguments: reverse: which sorts the list in the reverse order (descending) if True or in the regular order (ascending) if False …How to Create a List in Python. To create a list in Python, write a set of items within square brackets ( []) and separate each item with a comma. Items in a list can be any basic object type found in Python, including integers, strings, floating point values or boolean values. For example, to create a list named “z” that holds the integers ...Iterate through the list and use a if condition to check the matching names, if match found, click it. The problem is that clicking on a value like link [0].click () doesn't …Jan 28, 2022 ... Python allows us to create list subsets using slicing techniques. To create a list slice, we specify the index of the first and last items.Are you using python 2.7 or 3+? If your using 2.7 I recommending saving the array in a pickle file using the cPickle import. Otherwise you can look at Pickle for 3+. I know this doesn't answer your question but it would make it easier to read objects from hard drive. –Oct 10, 2019 · The function itertools.repeat doesn't actually create the list, it just creates an object that can be used to create a list if you wish! Let's try that again, but converting to a list: >>> timeit.timeit('list(itertools.repeat(0, 10))', 'import itertools', number = 1000000) 1.7508119747063233 So if you want a list, use [e] * n. You can create a list by placing all the items inside square brackets [ ], separated by commas. Lists can contain any type of object and this makes them very …Python has become one of the most popular programming languages in recent years. Whether you are a beginner or an experienced developer, there are numerous online courses available...Creating a linked list in Python. In this LinkedList class, we will use the Node class to create a linked list. In this class, we have an __init__ method that initializes the linked list with an empty head. Next, we have created an insertAtBegin() method to insert a node at the beginning of the linked list, an insertAtIndex() method to insert a node at the …A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.Jan 23, 2023 ... A Python list is created by adding elements in the square brackets [ ]. ... These lists can contain elements of different types. Creating a Python ...To make a deep copy of a list, in Python 2 or 3, use deepcopy in the copy module: import copy a_deep_copy = copy.deepcopy(a_list) ... Functionally, what we really want to do is make a new list that is based on the original. We don't need to make a copy first to do that, and we typically shouldn't.You can use the Python map () function along with the functools.reduce () function to compare the data items of two lists. When you use them in combination, the map () function applies the given function to every element and the reduce () function ensures that it applies the function in a consecutive manner.Jan 5, 2024 · One additional approach to convert a list to a string in Python is to use the str.format method. This method allows you to specify a string template, and then fill in the placeholder values with the elements in the list. For example: Python3. lst = ['Geeks', 'for', 'Geeks'] result = " {} {} {}".format(*lst) A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.Python can make alphabet list like Haskell? 6. Is There an Already Made Alphabet List In Python?-2. Is there a built in list in python or some package that has a list of the alphabet?-5. How can I create a list from a to z, and A to Z. 11. Is it possible to make a letter range in python? 9.Any nested or non-nested list/dictionary structure can be saved to a json file (as long as all the contents are serializable). Disadvantages: The data is stored in plain-text (ie it's uncompressed), which makes it a slow and …Iterate through the list and use a if condition to check the matching names, if match found, click it. The problem is that clicking on a value like link [0].click () doesn't …Sep 15, 2022 ... Trying to create a list of even numbers from a list but receiving an error. Here's what I have: def myfunc(*args): myfunc=[2,3,4,6 ...Jul 11, 2023 ... These include printing with the simple print() function, a loop, the join() method, list comprehension, enumeration, map() function, and the ...I need to operate on two separate infinite list of numbers, but could not find a way to generate, store and operate on it in python. Can any one please suggest me a way to handle infinite Arithmetic Progession or any series and how to operate on them considering the fact the minimal use of memory and time.You can sort a list in Python using the sort () method. The sort () method allows you to order items in a list. Here's the syntax: list.sort(reverse=True|False, key=sortFunction) The method accepts two optional arguments: reverse: which sorts the list in the reverse order (descending) if True or in the regular order (ascending) if False …Mar 12, 2024 · Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python. I'm new to python so pardon me if this is a silly question but I'm trying to return a list from a function. This is what I have so far but I'm getting nowhere. Can someone tell me what I'm doing wr...Now, list comprehension in Python does the same task and also makes the program more simple. List Comprehensions translate the traditional iteration approach using for loop into a simple formula hence making them easy to use. Below is the approach to iterate through a list, string, tuple, etc. using list comprehension in Python.Are you a Python developer tired of the hassle of setting up and maintaining a local development environment? Look no further. In this article, we will explore the benefits of swit...Jul 11, 2011 · It uses the 'Listoper' class from the listfun module that can be installed through pip. The appropriate function for your case would be: "listscale (a,b): Returns list of the product of scalar "a" with list "b" if "a" is scalar, or other way around" Code: !pip install listfun. from listfun import Listoper as lst. You can take a word and turn it into a list. Every single character that makes up that word becomes an individual and separate element inside the list. For example, let's take the text "Python". You can convert it to a list of characters, where each list item would be every character that makes up the string "Python".Now, list comprehension in Python does the same task and also makes the program more simple. List Comprehensions translate the traditional iteration approach using for loop into a simple formula hence making them easy to use. Below is the approach to iterate through a list, string, tuple, etc. using list comprehension in Python.Python is one of the most popular programming languages in the world. It is known for its simplicity and readability, making it an excellent choice for beginners who are eager to l... If you want to see the dependency with the length of the list n: Pure python. I tested for list length up to n=10000 and the behavior remains the same. So the integer multiplication method is the fastest with difference. Numpy. For lists with more than ~300 elements you should consider numpy. Benchmark code: Python offers a number of functional programming utilities even though it's primarily an object-oriented programming language. And the most notable one is the map() function. In this article, we'll explore what the map() function is and how to use it in your code. The map() function in Python The map()Tuple. Tuples are used to store multiple items in a single variable. Tuple is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Set, and Dictionary, all with different qualities and usage.. A tuple is a collection which is ordered and unchangeable.. Tuples are written with round brackets.Note that I demonstrated two different ways to make a copy of a list above: [:] and list(). The first, [:], is creating a slice (normally often used for getting just part of a list), which happens to contain the entire list, and thus is effectively a copy of the list. The second, list(), is using the actual list type constructor to create a new list which has contents equal to the first list.Python provides another composite data type called a dictionary, which is similar to a list in that it is a collection of objects.. Here’s what you’ll learn in this tutorial: You’ll cover the basic characteristics of Python dictionaries and learn how to access and manage dictionary data. Once you have finished this tutorial, you should have a good sense of when a dictionary …Some python adaptations include a high metabolism, the enlargement of organs during feeding and heat sensitive organs. It’s these heat sensitive organs that allow pythons to identi...May 3, 2020 ... This Video will help you to understand how to create a list in python • What is List? • How to use List • Assigning multiple values to List ...Dec 2, 2013 · writelines() needs a list of strings with line separators appended to them but your code is only giving it a list of integers. To make it work you'd need to use something like this: To make it work you'd need to use something like this: Scalars, Vector, and Matrices in Python. Here, I will explain what are scalars, vectors, and matrices in Python and how to create them. Scalars: A scalar in Python is one variable – for example, a string in a Python list but the list can take different values at different times.. In short, a list in Python that takes only one single value at a time will be …To create a list of lists in python, you can use the square brackets to store all the inner lists. For instance, if you have 5 lists and you want to create a list of lists …Feb 14, 2024 ... Using the list() function to initialize the list in Python ... It is another way to create an empty list without values in Python as the list() ...list() Syntax · list() Parameters · list() Return Value · Example 1: Create lists from string, tuple, and list · Example 2: Create lists from set and di...W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Method 3: Using While Loop. We can also use a while loop to replace values in the list. While loop does the same work as for loop. In the while loop first, we define a variable with value 0 and iterate over the list. If value matches to value that we want to replace then we replace it with the new value. Python3.Note that I demonstrated two different ways to make a copy of a list above: [:] and list(). The first, [:], is creating a slice (normally often used for getting just part of a list), which happens to contain the entire list, and thus is effectively a copy of the list. The second, list(), is using the actual list type constructor to create a new list which has contents equal to the first list.Dec 8, 2023 · I added two other ways to get the average of a list (which are relevant only for Python 3.8+). Here is the comparison that I made: ( thanx Robert for clarification, below is only in case of using listcomprehensions : )! another very important difference is that with round brackets we will have a generator and so the memory consumption is much lower in comparison to list with square brackets esspecially when you deal with big lists - generator will eat not only significantly less memory but …Note: To fully understand lists in Python you need to make sure you understand what mutable, ordered collection actually means.The fact that lists in Python are mutable means that a list in Python can be modified or changed after its creation. Elements can be added, removed, or updated within the list. On the other hand, …Classification: Python is a high-level object-oriented scripting language. Usage: Back-end web developers use Python to create web applications, analyze data, …Dec 17, 2022 ... a = [] b = ['initial value'] a.append(b) # Uses the actual b list. a.append(b.copy()) # Make a copy. a.append(b[ ...Method 1: Reverse in place with obj.reverse () If the goal is just to reverse the order of the items in an existing list, without looping over them or getting a copy to work with, use the <list>.reverse () function. Run this directly on a list object, and the order of all items will be reversed:A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using np.random.randint is the fastest option. Python list is an ordered sequence of items. In this article you will learn the different methods of creating a list, adding, modifying, and deleting elements in the list. Also, learn how to iterate the list and access the elements in the list in detail. Nested Lists and List Comprehension are also discussed in detail with examples.In Python 2, list was unnecessary since map returned a list: map(int, xs) Share. Improve this answer. Follow edited Sep 15, 2023 at 13:19. alper. 3,198 10 10 gold badges 56 56 silver badges 108 108 bronze badges. answered Sep 10, 2011 at 0:30. cheeken cheeken.Nov 29, 2023 · Below are the ways by which we can use list() function in Python: To create a list from a string; To create a list from a tuple; To create a list from set and dictionary; Taking user input as a list; Example 1: Using list() to Create a List from a String. In this example, we are using list() function to create a Python list from a string. I got here because I wanted to create a range between -10 and 10 in increments of 0.1 using list comprehension. Instead of doing an overly complicated function like most of the answers above I just did thisPython has a set of built-in methods that you can use on lists. Method. Description. append () Adds an element at the end of the list. clear () Removes all the elements from the list. copy () Returns a copy of the list.To start, here is a template that you may use to create a list in Python: list_name = [ 'item1', 'item2', 'item3', ....] You’ll now see how to apply this template in …Aug 16, 2013 · That is a list in Python 2.x and behaves mostly like a list in Python 3.x. If you are running Python 3 and need a list that you can modify, then use: Reversing a List in Python. Below are the approaches that we will cover in this article: Using the slicing technique. Reversing list by swapping present and last numbers at a time. Using the reversed () and reverse () built-in function. Using a two-pointer approach. Using the insert () function. Using list comprehension.NumPy is the fundamental Python library for numerical computing. Its most important type is an array type called ndarray.NumPy offers a lot of array creation routines for different …Program to cyclically rotate an array by one in Python | List Slicing; Python | Check if list contains all unique elements; Python Program to Accessing index and value in list; Python | Print all the common elements of two lists; Python | Cloning or Copying a list; Python | Ways to shuffle a list; How to count unique values inside a listJul 17, 2021 · The fact that I can type does not mean that I can "python", I really like gnibbler's answer over for-messy-things. Thanks everyone for your answers and -- keep things simple, special thanks to gnibbler. You can create a list by placing all the items inside square brackets [ ], separated by commas. Lists can contain any type of object and this makes them very …Python is a popular programming language used by developers across the globe. Whether you are a beginner or an experienced programmer, installing Python is often one of the first s...You can sort a list in Python using the sort () method. The sort () method allows you to order items in a list. Here's the syntax: list.sort(reverse=True|False, key=sortFunction) The method accepts two optional arguments: reverse: which sorts the list in the reverse order (descending) if True or in the regular order (ascending) if False … Be aware, that in Python 3.x map() does not return a list (so you would need to do something like this: new_list = list(map(my_func, old_list))). Filling other list using simple for ... in loop. Alternatively you could use simple loop - it is still valid and Pythonic: new_list = [] for item in old_list: new_list.append(item * 10) Generators 1) there's no reason not to do my_list = sorted([int(raw_input('Please type a number')) for _ in xrange(10)) versus typing extra stuff. 2) you have a list called sorted_list but you don't actually sort it 3) There's nothing in the question asking about filtering out odd numbers only 4) What does this provide that the previous answers 5 year ago didn't …4.Return the concatenated list as the result of the function. 5.Define a list called my_list containing the integers 1 through 5. 6.Call the rotate_list function with my_list and the argument 2, and assign the result to a variable called rotated_list. 7.Print the value of rotated_list to the console.. Feb 16, 2023 · How to Create a List in Python. You cIn Python, “strip” is a method that eliminates If you only need to iterate through it on the fly then the chain example is probably better.) It works by pre-allocating a list of the final size and copying the parts in by slice (which is a lower-level block copy than any of the iterator methods): def join(a): """Joins a sequence of sequences into a single sequence. Ok there is a file which has different words in 'em. I have do Python is a versatile programming language that is widely used for its simplicity and readability. Whether you are a beginner or an experienced developer, mini projects in Python c... If you’re on the search for a python that’s just as b...
Continue Reading