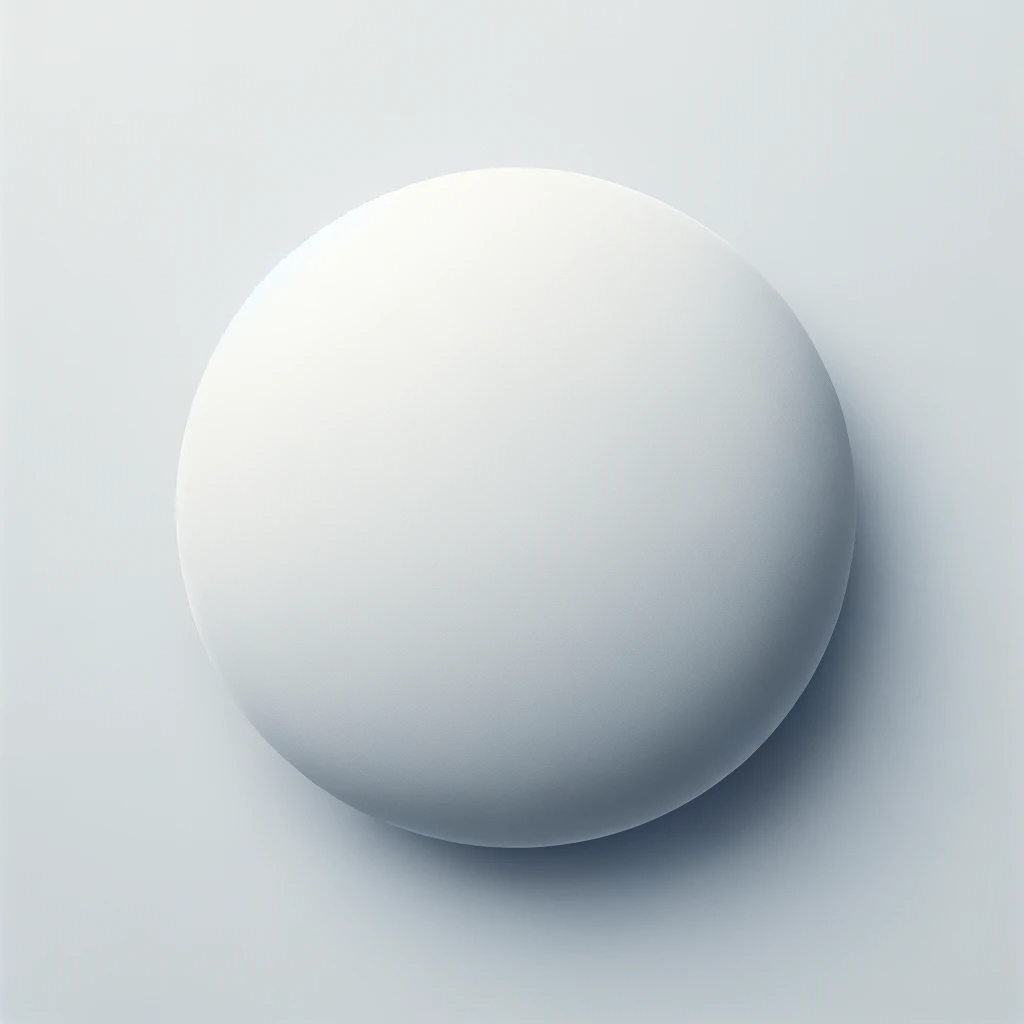
Nov 3, 2023 · Python String Methods for Performing Queries on a String; Python String Methods for Returning a Boolean Value; Python Bytes Methods that Return a String; Conclusion; Challenge; Textual content Sequence Sort and the str Class. Strings are one in all Python’s built-in sorts. Which means string information sorts, like different sorts, are ... The string methods. Python has a set of built-in methods that you can use on strings. isdigit () The method checks whether the strings consist only of digits and returns true …Python String Methods: Overview. Python String Methods that Return a Modified Version of a String. Python String Methods for Joining and Splitting Strings. …String operations #. Return element-wise string concatenation for two arrays of str or unicode. Return (a * i), that is string multiple concatenation, element-wise. Return (a % i), that is pre-Python 2.6 string formatting (interpolation), element-wise for a pair of array_likes of str or unicode. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python has a set of built-in methods that you can use on dictionaries. Method. Description. clear () Removes all the elements from the dictionary. copy () Returns a copy of the dictionary. fromkeys () Returns a dictionary with the specified keys and value.The idea behind f-strings is to make string interpolation simpler. To create an f-string, prefix the string with the letter “ f ”. The string itself can be formatted in much the same way that you would with str. format(). F-strings provide a concise and convenient way to embed Python expressions inside string literals for formatting.The Python String len () method is used to retrieve the length of the string. A string is a collection of characters and the length of a string is the number of characters (Unicode values) in it. The len () method determines how many characters are there in the string including punctuation, space, and all type of special characters.One is (start, length) and the other is (start, end). Python's (start, end+1) is admittedly unusual, but fits well with the way other things in Python work. A common way to achieve this is by string slicing. MyString [a:b] gives you a substring from index a to (b - 1).Python String Methods: replace() Method . There are also some python string methods used to find and replace any string in a given string. Python replace() function is used to find and replace any string. With this replace() function, we can use three parameters. The first one is the replaced Word, the second one is the new Word and the last one is the number of change.Your question is about Python 2.7, but it is worth note that from Python 3.6 onward we can use f-strings: place = 'world' f'hallo {place}' 'hallo world' This f prefix, called a formatted string literal or f-string, is described in the documentation on lexical analysisA Square Business Debit Card can help business owners get an immediate grip on their cash flow and provide peace of mind when unexpected expenses arise. The pandemic has had a prof... Built-in String Functions in Python. The following table lists all the functions that can be used with the string type in Python 3. Method. Description. capitalize () Returns the copy of the string with its first character capitalized and the rest of the letters are in lowercased. casefold () Returns a lowered case string. Python provides string methods that allows us to chop a string up according to delimiters that we can specify. In other words, we can tell Python to look for a certain substring within our target string, and split the target string up around that sub-string. It does that by returning a list of the resulting sub-strings (minus the delimiters).2) Concatenating strings using the + operator. A straightforward way to concatenate multiple strings into one is to use the + operator: s = 'String' + ' Concatenation' print (s) Code language: PHP (php) And the + operator works for both literal strings and string variables. For example: s1 = 'String'. s2 = s1 + ' Concatenation' print (s2) Code ...Python Overview Python Built-in Functions Python String Methods Python List Methods Python Dictionary Methods Python Tuple Methods Python Set Methods Python File Methods Python Keywords Python Exceptions Python Glossary Module Reference Random Module Requests Module Statistics Module Math Module cMath Module Python How To Remove List …The replace() method does not change the string it is called on. The replace() method returns a new string. The replace() method replaces only the first match. If you want to replace all matches, use a regular expression with the /g flag set. See examples below.Does Python have a string contains substring method? 99% of use cases will be covered using the keyword, in, which returns True or False: 'substring' in any_string For the use case of getting the index, use str.find (which returns -1 on failure, and has optional positional arguments):. start = 0 stop = len(any_string) any_string.find('substring', start, …Aug 31, 2016 · One is (start, length) and the other is (start, end). Python's (start, end+1) is admittedly unusual, but fits well with the way other things in Python work. A common way to achieve this is by string slicing. MyString [a:b] gives you a substring from index a to (b - 1). Use built-in Python functions with characters and strings. Use methods to manipulate and modify string data. You’ll also be introduced to two other Python objects used to represent raw byte data: the bytes and bytearray types. Take the Quiz: Test your knowledge with our interactive “Python Strings and Character Data” quiz.Python has a set of built-in methods that you can use on lists/arrays. Add the elements of a list (or any iterable), to the end of the current list. Returns the index of the first element with the specified value. Note: Python does not have built-in support for Arrays, but Python Lists can be used instead.On this article, we’ll cowl helpful Python string strategies for manipulating string (str) objects — corresponding to becoming a member of, splitting and capitalizing. Every methodology described on this article will embrace a proof with a related instance. We’ll additionally finish with slightly problem you need to attempt to assess how a lot …W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Mar 7, 2023 ... Python String Functions | Python String Methods | Functions and Methods in Python | SuMyPyLab Learns the basics of Python String ...The Python String len () method is used to retrieve the length of the string. A string is a collection of characters and the length of a string is the number of characters (Unicode values) in it. The len () method determines how many characters are there in the string including punctuation, space, and all type of special characters.Python Strings Slicing Strings Modify Strings Concatenate Strings Format Strings Escape Characters String Methods String Exercises. ... Specify the start index and the end index, separated by a colon, to return a part of the string. Example. Get the characters from position 2 to position 5 (not included): ...The strptime () class method takes two arguments: string (that be converted to datetime) format code. Based on the string and format code used, the method returns its equivalent datetime object. In the above example: Here, %d - Represents the day of the month. Example: 01, 02, ..., 31. %B - Month's name in full.The str () function in Python is considered a substitute for tostring () method offered by other programming languages. It is a built-in function to convert objects into strings. For instance, consider a variable holding an integer value, say 10. var1 = 10 print ( type (var1)) The type () statement will help us determine that the variable is an ...31 ESSENTIAL Python String Methods. Patrick Loeber. 257K subscribers. Subscribed. 3K. 79K views 2 years ago #Python. Strings are an essential data type in …The encode() method encodes the string, using the specified encoding. If no encoding is specified, UTF-8 will be used. Syntax. string.encode(encoding=encoding, errors=errors) Parameter Values. Parameter Description; encoding: Optional. A String specifying the encoding to use. Default is UTF-8:Python Tutorials. Python is an interpreted and general-purpose programming language that emphasizes code readability with its use of significant indentation. Its object-oriented approach helps programmers write clear, logical code for small and large-scale projects. Python comes with a comprehensive standard library and has a wide range of ...Python String partition() method splits the string at the first occurrence of the separator and returns a tuple containing the part before the separator, the separator, and the part after the separator. Here, the separator is a string that is given as the argument. Example: Python3. str = "I love Geeks for geeks" print(str.partition("for")) Output: ('I love Geeks ', 'for', ' …The split () method takes a maximum of 2 parameters: separator (optional) - Specifies the delimiter used to split the string. If not provided, whitespace is used as the default delimiter. maxsplit (optional) - Determines the maximum number of splits. If not provided, the default value is -1, which means there is no limit on the number of splits. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python has a built-in string class named str . Python strings are "immutable" which means they cannot be changed after they are created. For string manipulation ...Method: In Python, we can use the function split() to split a string and join() to join a string. the split() method in Python split a string into a list of strings after breaking the given string by the specified separator. Python String join() method is a string method and returns a string in which the elements of the sequence have been …A classical acoustic guitar has six strings. There are variations in guitar configurations for creating different sounds, including the electric four-string bass guitar and the 12-...Python has had awesome string formatters for many years but the documentation on them is far too theoretic and technical. With this site we try to show you the most common use-cases covered by the old and new style string formatting API with practical examples.. All examples on this page work out of the box with with Python 2.7, 3.2, 3.3, 3.4, and 3.5 … The String to Reverse. txt = "Hello World" [::-1] print(txt) Create a slice that starts at the end of the string, and moves backwards. In this particular example, the slice statement [::-1] means start at the end of the string and end at position 0, move with the step -1, negative one, which means one step backwards. Output: False True True True. Time complexity: O(n), where n is the length of the string being searched for. Auxiliary space: O(1), as the method endswith() only requires a constant amount of memory to store the variables text, result, and the string being searched for. Python endswith() With start and end Parameters. we shall add two extra parameters, the …The Python String replace () method replaces all occurrences of one substring in a string with another substring. This method is used to create another string by replacing some parts of the original string, whose gist might remain unmodified. For example, in real-time applications, this method can be used to replace multiple same spelling ...The str () function in Python is considered a substitute for tostring () method offered by other programming languages. It is a built-in function to convert objects into strings. For instance, consider a variable holding an integer value, say 10. var1 = 10 print ( type (var1)) The type () statement will help us determine that the variable is an ...Strings are an essential data type in Python that are used in nearly every application. In this tutorial we learn about the 31 most important built-in string... W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Strings in Python are represented using the built-in str type/class. This class defines methods which can be used to manipulate or extract information from string instances. …The Python String replace () method replaces all occurrences of one substring in a string with another substring. This method is used to create another string by replacing some parts of the original string, whose gist might remain unmodified. For example, in real-time applications, this method can be used to replace multiple same spelling ...Repeat String. In the above example, you can observe that the string "PFB "is repeated 5 times when we multiply it by 5.. Replace Substring in a String in Python. You can replace a substring with another substring using the replace() method in Python. The replace() method, when invoked on a string, takes the substring to replaced as its first …The syntax of lower() method is: string.lower() lower() Parameters() lower() method doesn't take any parameters. lower() Return value. lower() method returns the lowercase string from the given string. It converts all uppercase characters to lowercase.Collections of text in Python are called strings. Special functions called string methods are used to manipulate strings. There are string methods for changing a string from lowercase to uppercase, removing …Definition and Usage. The find () method finds the first occurrence of the specified value. The find () method returns -1 if the value is not found. The find () method is almost the same as the index () method, the only difference is that the index () method raises an exception if the value is not found. (See example below) W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. The ljust() method will left align the string, using a specified character (space is default) as the fill character. Syntax. string.ljust(length, character) Parameter Values. Parameter Description; length: Required. The length of the returned string: character: Optional. A character to fill the missing space (to the right of the string).The Python String replace () method replaces all occurrences of one substring in a string with another substring. This method is used to create another string by replacing some parts of the original string, whose gist might remain unmodified. For example, in real-time applications, this method can be used to replace multiple same spelling ...Aug 17, 2019 ... In this video, I have explained what strings in python are and how to use them to write effective python programs.Join Sets element into a String using join() method. In this example, we are using a Python set to join the string. ... File "Solution.py", line 4, in <module> string = '_'.join(dic) TypeError: sequence item 0: expected string, int found Joining a list of Strings with a Custom Separator using Join()Python Tutorials. Python is an interpreted and general-purpose programming language that emphasizes code readability with its use of significant indentation. Its object-oriented approach helps programmers write clear, logical code for small and large-scale projects. Python comes with a comprehensive standard library and has a wide range of ...Add a comment. 9. def kill_char(string, n): # n = position of which character you want to remove. begin = string[:n] # from beginning to n (n not included) end = string[n+1:] # n+1 through end of string. return begin + end. print kill_char("EXAMPLE", 3) # "M" removed. I have seen this somewhere here. Share. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Converting Between Strings and Lists. Methods in this group convert between a string and some composite data type by either pasting objects together to make a string, or by breaking a string up into pieces. These methods operate on or return iterables, the general Python term for a sequential collection of objects. You will explore the inner .... Run Python code live in your browser. Write and run code in 50+ lanThe syntax of lower() method is: string.lower() lower() Parameters() The Growing String Method (GSM) is a reaction path (RP) and transition state (TS) finding tool. GSM is utilized in two main fashions, double-ended (DE) and single-ended (SE). DE requires a reactant and product pair, wheras SE only requires a reactant and a driving coorindate. The driving coordinate are internal coordinates (angles, bonds, and ... W3Schools offers free online tutorials, refer Run Python code live in your browser. Write and run code in 50+ languages online with Replit, a powerful IDE, compiler, & interpreter.Dec 27, 2022 ... Find and Replace · encode(): it encodes the string using a specified encoding scheme. · find(): it returns the lowest index of the specified ... Using casefold() Method ; String with Case-Insen...
Continue Reading