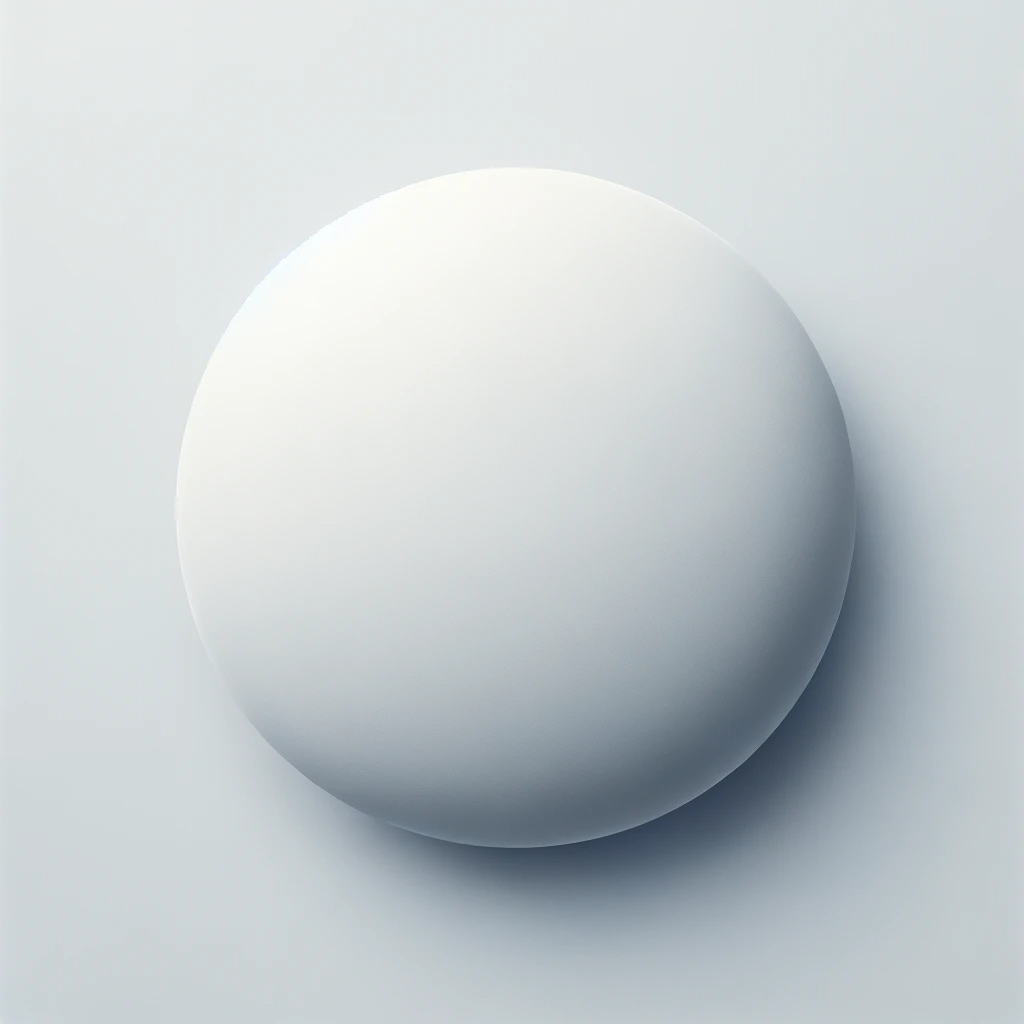
Jul 19, 2023 · Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, comprehensions, and functional tools. Multiply all numbers in the list using numpy.prod () We can use numpy.prod () from import numpy to get the multiplication of all the numbers in the list. It returns an integer or a float value depending on the multiplication result. Example : In this example the below code uses `numpy.prod ()` to find the product of elements in lists ` [1, 2, 3 ...You can also pass in built-in Python functions. For example if you had a list of strings, you can easily create a new list with the length of each string in the list. org_list = ["Hello", "world", "freecodecamp"] fin_list = list(map(len, org_list)) print(fin_list) # [5, 5, 12] How to Use Functions with Multiple Iterables in PythonExamining the first ten years of Stack Overflow questions, shows that Python is ascendant. Imagine you are trying to solve a problem at work and you get stuck. What do you do? Mayb...Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, …Pickling - is the process whereby a Python object hierarchy is converted into a byte stream, and Unpickling - is the inverse operation, whereby a byte stream is converted back into an object hierarchy. Pickling (and unpickling) is alternatively known as serialization, marshalling, or flattening. import pickle. data1 = {'a': [1, 2.0, 3, 4+6j],Learn how to define, manipulate, and use lists and tuples in Python, two of the most versatile and useful data types. See examples of ordered, mutable, and dynamic …Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... The sample() method returns a list with a specified number of randomly selected items from a sequence. Note: This method does not change the original sequence. Syntax.When we find the target element, we calculate the negative index using the formula - (i+1) and break out of the loop. If the element is not found in the list, the index variable remains at its initial value of -1. Finally, we print the negative index. Python3. test_list = [5, 7, 8, 2, 3, 5, 1]W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.A Python list is a collection of items that are ordered and changeable. Lists are written with square brackets, and the items are separated by commas. Here is an example of a …Example 1: Create lists from string, tuple, and list. # empty list print(list()) # vowel string . vowel_string = 'aeiou' print (list(vowel_string)) # vowel tuple . vowel_tuple = ('a', 'e', 'i', …In the following example, we will define a list with different datatypes. Python Program. list1 = ["Apple", 54, 87.36, True] print(list1) Run Code Copy. Output. ['Apple', 54, 87.36, …Python List Exercises, Practice and Solution - Contains 280 Python list exercises with solutions for beginners to advanced programmers. These exercises cover various topics such as summing and multiplying items, finding large and small numbers, removing duplicates, checking emptiness, cloning or copying lists, generating 3D arrays, …Python List - Learn By Example. A list is a sequence of values (similar to an array in other programming languages but more versatile) The values in a list are called items or …Python provides another composite data type called a dictionary, which is similar to a list in that it is a collection of objects.. Here’s what you’ll learn in this tutorial: You’ll cover the basic characteristics of Python dictionaries and learn how to access and manage dictionary data. Once you have finished this tutorial, you should have a good sense of when a dictionary …In this tutorial, we will learn about the Python List extend() method with the help of examples. Courses Tutorials Examples . Try Programiz PRO. Course Index Explore Programiz ... Example 2: Add Items from Other Iterables languages = ['French'] languages_tuple = ('Spanish', 'Portuguese') # add items of the tuple to the languages list …W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ...An exception is an unexpected event that occurs during program execution. For example, divide_by_zero = 7 / 0. The above code causes an exception as it is not possible to divide a number by 0. Let's learn about Python Exceptions in detail.List comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list. Example: Based on a list of fruits, you want a new list, containing only the fruits with the letter "a" in the name. Without list comprehension you will have to write a for statement with a conditional test inside:An iterator in Python is an object that is used to iterate over iterable objects like lists, tuples, dicts, and sets. The Python iterators object is initialized using the iter () method. It uses the next () method for iteration. __iter__ (): The iter () method is called for the initialization of an iterator. This returns an iterator object.A list of odd numbers is a list of numbers that all have a remainder of 1 when divided by 2. The following is an example of a list of odd numbers: 1, 3, 5, 7, 9, 11, 13 15, 17, 19 ...W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.In Python, there are two ways to add elements to a list: extend () and append (). However, these two methods serve quite different functions. In append () we add a single element to the end of a list. In extend () we add multiple elements to a list. The supplied element is added as a single item at the end of the initial list by the append ...In the above example, the personal_info is a list of tuples where the get_age function is defined as a key function. Here, the list is sorted based on the age (i.e., second element) of each tuple. Sort Example With Both Parameters We can use both key and reverse parameters for sorting the list. For example, words = ['python', 'is', 'awesome ...C# List. List<T> is a class that contains multiple objects of the same data type that can be accessed using an index. For example, // list containing integer values. List<int> number = new List<int>() { 1, 2, 3 }; Here, number is a List containing integer values ( 1, 2 and 3 ).Dec 17, 2019 · What Is a List in Python? A list is a data structure that's built into Python and holds a collection of items. Lists have a number of important characteristics: List items are enclosed in square brackets, like this [item1, item2, item3]. Lists are ordered – i.e. the items in the list appear in a specific order. This enables us to use an index ... Mar 1, 2023 · A call stack is an ordered list of functions that are currently being executed. For example, you might call function A, which calls function B, which calls function C. We now have a call stack consisting of A, B, and C. When C raises an exception, Python will look for an exception handler in this call stack, going backward from end to start. list clear () is an in-built function in Python, that is used to delete every element from a list. It can make any list into a null list and should be used with caution. Unmute. ×. It is one of the most basic and known list operations. It is very useful for re-using a list, as you can delete every element from that list. Examples. 1. Sort elements in a list. Following is simple example program where we use sorted () built-in function to sort a list of numbers. We provide this list of numbers as iterable, and not provide key and reverse values as these are optional parameters. Python Program. nums = [2, 8, 1, 6, 3, 7, 4, 9] In Python, we use = operator to create a copy of an object. You may think that this creates a new object; it doesn't. It only creates a new variable that shares the reference of the original object. Let's take an example where we create a list named old_list and pass an object reference to new_list using = operator. Example 1: Copy using = operator13 Jul 2023 ... get() function. You can access an item in the list by referring to its index number. Python list index starts from 0. The following are examples ...What is Python Nested List? A list can contain any sort object, even another list (sublist), which in turn can contain sublists themselves, and so on. This is known as nested list.. You can use them to arrange data into hierarchical structures. Create a Nested List. A nested list is created by placing a comma-separated sequence of sublists."Guardians of the Glades" promises all the drama of "Keeping Up With the Kardashians" with none of the guilt: It's about nature! Dusty “the Wildman” Crum is a freelance snake hunte...When we find the target element, we calculate the negative index using the formula - (i+1) and break out of the loop. If the element is not found in the list, the index variable remains at its initial value of -1. Finally, we print the negative index. Python3. test_list = [5, 7, 8, 2, 3, 5, 1]Python has a set of built-in methods that you can use on lists. Method. Description. append () Adds an element at the end of the list. clear () Removes all the elements from the list. copy () Returns a copy of the list.Python Lists. A Python list is a built-in data structure that holds a collection of items. To understand how lists work, let’s go straight to the example. We want to create a list that includes names of the US presidents elected in the last six presidential elections. List elements should be enclosed in square brackets [] andThe benefit of this solution is that all arrays will be at most different by 1 in size. The accepted answer could have the last chunk a lot shorter.Learn how to create, access, modify, sort, and use lists in Python. This tutorial covers the basics and advanced features of lists, such as slicing, copying, …Python lists have a built-in list.sort() method that modifies the list in-place. There is also a sorted() built-in function that builds a new sorted list from an iterable. In this document, we explore the various techniques for sorting data using Python. Sorting Basics¶ A simple ascending sort is very easy: just call the sorted() function.Python is a versatile programming language that is widely used for its simplicity and readability. Whether you are a beginner or an experienced developer, mini projects in Python c... Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. Or at least use them as context clues to learn more about the property. When it comes to your hard-earned vacation, a rental property can make or break the trip. For example, you m...3 days ago · List comprehensions provide a concise way to create lists. Common applications are to make new lists where each element is the result of some operations applied to each member of another sequence or iterable, or to create a subsequence of those elements that satisfy a certain condition. For example, assume we want to create a list of squares, like: The benefit of this solution is that all arrays will be at most different by 1 in size. The accepted answer could have the last chunk a lot shorter.Python has a set of built-in methods that you can use on lists. Method. Description. append () Adds an element at the end of the list. clear () Removes all the elements from the list. copy () Returns a copy of the list.Pickling - is the process whereby a Python object hierarchy is converted into a byte stream, and Unpickling - is the inverse operation, whereby a byte stream is converted back into an object hierarchy. Pickling (and unpickling) is alternatively known as serialization, marshalling, or flattening. import pickle. data1 = {'a': [1, 2.0, 3, 4+6j], W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python List of Lists. Python List of Lists is a Python list containing elements that are Lists. We know that a Python List can contain elements of any type. So, if we assign Python lists for these elements, we get a Python List of Lists. Python List of Lists is similar to a two dimensional array. Inner lists can have different sizes. Create ...The list() returns a list object from an iterable passed as arguement with all elements.Python is a versatile programming language that is widely used for its simplicity and readability. Whether you are a beginner or an experienced developer, mini projects in Python c...Python’s enumerate () has one additional argument that you can use to control the starting value of the count. By default, the starting value is 0 because Python sequence types are indexed starting with zero. In other words, when you want to retrieve the first element of a list, you use index 0: Python.An iterator in Python is an object that is used to iterate over iterable objects like lists, tuples, dicts, and sets. The Python iterators object is initialized using the iter () method. It uses the next () method for iteration. __iter__ (): The iter () method is called for the initialization of an iterator. This returns an iterator object.random.sample () randomly samples multiple elements from a list without replacement, taking a list as the first argument and the number of elements to retrieve …Learn everything you need to know about Python lists, one of the most used data structures in Python. See how to create, access, modify, sort, slice, reverse, and …What is Python Nested List? A list can contain any sort object, even another list (sublist), which in turn can contain sublists themselves, and so on. This is known as nested list.. You can use them to arrange data into hierarchical structures. Create a Nested List. A nested list is created by placing a comma-separated sequence of sublists.W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Apr 27, 2021 · 🔹 List and Dictionary Comprehension in Python. A really nice feature of Python that you should know about is list and dictionary comprehension. This is just a way to create lists and dictionaries more compactly. List Comprehension in Python. The syntax used to define list comprehensions usually follows one of these four patterns: @RK1 glad it works :-) list is a keyword in python, so it's usually not a good idea to call your parameter a keyword, as it will "shadow" it. So I use the word array, as this is the actual functionality of the list that I'm using - their array like manner. ... Example: def calcperm(arr, size, dupes): where dupes are the allowed numbers of ...Algorithm. The bubble sort algorithm works as follows. Step 1) Get the total number of elements. Get the total number of items in the given list. Step 2) Determine the number of outer passes (n – 1) to be done. Its length is list minus one. Step 3) Perform inner passes (n – 1) times for outer pass 1.Python’s enumerate () has one additional argument that you can use to control the starting value of the count. By default, the starting value is 0 because Python sequence types are indexed starting with zero. In other words, when you want to retrieve the first element of a list, you use index 0: Python.If you are a Python programmer, it is quite likely that you have experience in shell scripting. It is not uncommon to face a task that seems trivial to solve with a shell command. ...How to Create a List in Python. To create a list in Python, write a set of items within square brackets ( []) and separate each item with a comma. Items in a list can be any basic object type found in Python, including integers, strings, floating point values or boolean values. For example, to create a list named “z” that holds the integers ...In this tutorial, we will learn about the Python List extend() method with the help of examples. Courses Tutorials Examples . Try Programiz PRO. Course Index Explore Programiz ... Example 2: Add Items from Other Iterables languages = ['French'] languages_tuple = ('Spanish', 'Portuguese') # add items of the tuple to the languages list …Neptyne, a startup building a Python-powered spreadsheet platform, has raised $2 million in a pre-seed venture round. Douwe Osinga and Jack Amadeo were working together at Sidewalk...Second, iterate over the elements of the scores list. If the element is greater than or equal to 70, add it to the filtered list. Third, show the filtered list to the screen. Python has a built-in function called filter() that allows you to filter a list (or a tuple) in a more beautiful way. The following shows the syntax of the filter() function:List comprehension along with zip() function is used to convert the tuples to list and create a list of tuples. Python iter() function is used to iterate an element of an object at a time. The ‘number‘ would specify the number of elements to be clubbed into a single tuple to form a list. Example 1:The functions from the itertools module return iterators. All you need to do to convert these into lists is call list() on the result.. However, since you will need to call itertools.combinations three separate times (once for each different length), you can just use list.extend to add all elements of the iterator to your final list.. Try the following:An exception is an unexpected event that occurs during program execution. For example, divide_by_zero = 7 / 0. The above code causes an exception as it is not possible to divide a number by 0. Let's learn about Python Exceptions in detail.A list [/news/how-to-make-a-list-in-python-declare-lists-in-python-example/] is one of the data structures in Python that you can use to store a collection of variables. In some cases, you have to iterate through and perform an operation on the elements of a list. But you can't loop/iterate through a list if it hasClone or Copy a List. When you execute new_List = old_List, you don’t actually have two lists. The assignment just copies the reference to the list, not the actual list. So, both new_List and old_List refer to the same list after the assignment. You can use slicing operator to actually copy the list (also known as a shallow copy).In this example, running python program.py -s hello world will produce Concatenated strings: hello world. Example 3: Using an integer for a fixed number of values. import argparse # Initialize the ArgumentParser parser = argparse.ArgumentParser(description="A program to demonstrate nargs with a fixed … The items of a list are in order, and we can access individual items of a list using indexes. For example, cars = ['BMW', 'Tesla', 'Ford'] # access the first item print (cars [0]) # BMW # access the third item print (cars [2]) # Ford. In Python, indexing starts from 0. Meaning, the index of the first item is 0. the index of the second item is 1. Python List - Learn By Example. A list is a sequence of values (similar to an array in other programming languages but more versatile) The values in a list are called items or …Python Tuple Data Type. Tuple is an ordered sequence of items same as a list. The only difference is that tuples are immutable. Tuples once created cannot be modified. In Python, we use the parentheses to store items of a tuple. For example, product = ('Xbox', 499.99) Here, product is a tuple with a string value Xbox and integer value 499.99."Guardians of the Glades" promises all the drama of "Keeping Up With the Kardashians" with none of the guilt: It's about nature! Dusty “the Wildman” Crum is a freelance snake hunte...Sep 8, 2023 · Basics of Python Lists. A list in Python is a mutable, ordered collection of elements. The elements can be of various data types such as integers, floats, strings, or even other data structures like dictionaries and sets. The flexibility and dynamic nature of lists make them an invaluable asset when working with data in Python. Dec 1, 2023 · List insert () method in Python is very useful to insert an element in a list. What makes it different from append () is that the list insert () function can add the value at any position in a list, whereas the append function is limited to adding values at the end. It is used in editing lists with huge amount of data, as inserting any missed ... W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.Clone or Copy a List. When you execute new_List = old_List, you don’t actually have two lists. The assignment just copies the reference to the list, not the actual list. So, both new_List and old_List refer to the same list after the assignment. You can use slicing operator to actually copy the list (also known as a shallow copy).In this example, we created a two-dimensional list called data containing nested lists. The DataFrame() function converts the 2-D list to a DataFrame. Each nested list behaves like a row of data in the DataFrame. The columns argument provides a name to each column of the DataFrame. Note: We can also create a DataFrame using NumPy array in a ... The items of a list are in order, and we can access individual items of a list using indexes. For example, cars = ['BMW', 'Tesla', 'Ford'] # access the first item print (cars [0]) # BMW # access the third item print (cars [2]) # Ford. In Python, indexing starts from 0. Meaning, the index of the first item is 0. the index of the second item is 1. 13 Jul 2023 ... get() function. You can access an item in the list by referring to its index number. Python list index starts from 0. The following are examples .... A list, a type of sequence data, is used to store the collIn this tutorial, we will learn about the Python Li "Guardians of the Glades" promises all the drama of "Keeping Up With the Kardashians" with none of the guilt: It's about nature! Dusty “the Wildman” Crum is a freelance snake hunte...Use the random.choices () function to select multiple random items from a sequence with repetition. For example, You have a list of names, and you want to choose random four names from it, and it’s okay for you if one of the names repeats. A random.choices () function introduced in Python 3.6. 23 Jul 2019 ... Python List · The list is a mutable sequence Python’s enumerate () has one additional argument that you can use to control the starting value of the count. By default, the starting value is 0 because Python sequence types are indexed starting with zero. In other words, when you want to retrieve the first element of a list, you use index 0: Python. Removes all the elements from the list. copy () Returns a copy of the list. count () Returns the number of elements with the specified value. extend () Add the elements of a list (or any iterable), to the end of the current list. index () Returns the index of the first element with the specified value. An iterator in Python is an object that is used to iterate o...
Continue Reading