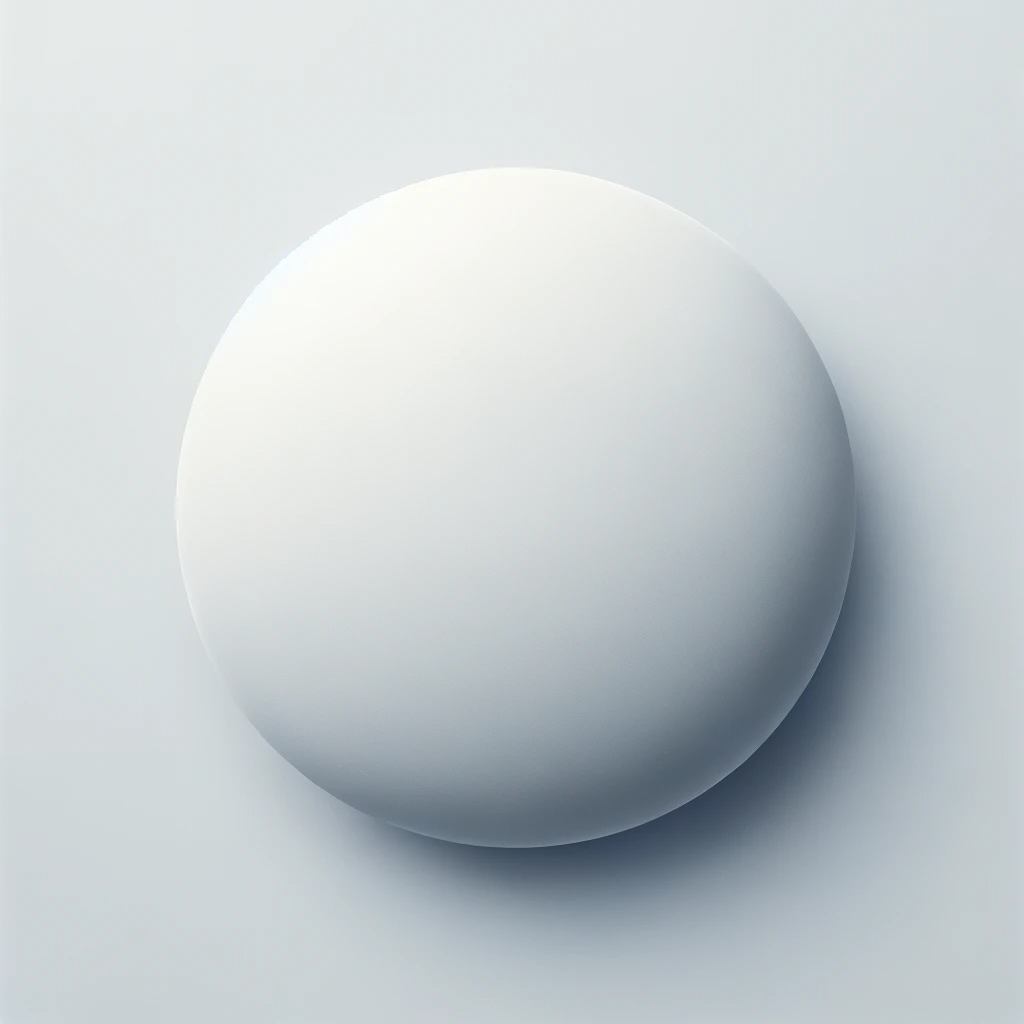
Rule 1: Don't do it. Rule 2 (for experts only): Don't do it yet. And the Knuth rule: "Premature optimization is the root of all evil." The more useful rules …The first step to solve a quadratic equation is to calculate the discriminant. Using simple formula: D = b2– 4ac. we can solve for discriminant and get some value. Next, if the value is: positive, then the equation has two solutions. zero, then the equation has one repeated solution. negative, then the equation has no solutions.Following the previous article on modeling and solving an optimization problem in Python using several “interfaces” (), in this article, I try to provide a comprehensive review of open-source (OS), free, free & open-source (FOSS), and commercial “solvers,” which are usually used for specific types of problems and coded …Optimization is the problem of finding a set of inputs to an objective function that results in a maximum or minimum function evaluation. It is the challenging problem that underlies many machine learning algorithms, from fitting logistic regression models to training artificial neural networks. There are perhaps hundreds of popular optimization …The homepage for Pyomo, an extensible Python-based open-source optimization modeling language for linear programming, nonlinear programming, ...RSOME (Robust Stochastic Optimization Made Easy) is an open-source Python package for generic modeling of optimization problems (subject to uncertainty). Models in RSOME are constructed by variables, constraints, and expressions that are formatted as N-dimensional arrays. These arrays are consistent with the NumPy library …1. Redis would be a great option here if you have the option to use it on a shared host - similar to memcached, but optimised for data structures. Redis also supports python bindings. I use it on a day to day basis for number crunching but also in production systems as a datastore and cannot recommend it highly …May 25, 2022 · Newton’s method for optimization is a particular case of a descent method. With “ f′′ (xk ) ” being the derivative of the derivative of “ f” evaluated at iteration “ k”. Consider ... To better understand the Peephole optimization technique, let’s start with how the Python code is executed. Initially the code is written to a standard file, then you can run the command “python -m compileall <filename>”and get the same file in *.pyc format which is the result of the optimization. <Peephole> is a code …scipy.optimize.fsolve# scipy.optimize. fsolve (func, x0, args = (), fprime = None, full_output = 0, col_deriv = 0, xtol = 1.49012e-08, maxfev = 0, band = None, epsfcn = None, factor = 100, diag = None) [source] # Find the roots of a function. Return the roots of the (non-linear) equations defined by func(x) = 0 given a starting estimate ...You were correct that my likelihood function was wrong, not the code. Using a formula I found on wikipedia I adjusted the code to: m = parameters[0] b = parameters[1] sigma = parameters[2] for i in np.arange(0, len(x)): y_exp = m * x + b. L = (len(x)/2 * np.log(2 * np.pi) + len(x)/2 * np.log(sigma ** 2) + 1 /. (2 * sigma ** 2) * sum((y - y_exp ...Optimization-algorithms is a Python library that contains useful algorithms for several complex problems such as partitioning, floor planning, scheduling. This library will provide many implementations for many optimization algorithms. This library is organized in a problem-wise structure. For example, there are many problems such as graph ... Optimization happens everywhere. Machine learning is one example of such and gradient descent is probably the most famous algorithm for performing optimization. Optimization means to find the best value of some function or model. That can be the maximum or the minimum according to some metric. Using clear explanations, standard Python libraries ... Oct 12, 2021 · Optimization refers to a procedure for finding the input parameters or arguments to a function that result in the minimum or maximum output of the function. The most common type of optimization problems encountered in machine learning are continuous function optimization, where the input arguments to the function are real-valued numeric values ... Oct 24, 2015 · The minimize function provides a common interface to unconstrained and constrained minimization algorithms for multivariate scalar functions in scipy.optimize. To demonstrate the minimization function consider the problem of minimizing the Rosenbrock function of N variables: f(x) = ∑i=1N−1 100xi −x2i−1) The minimum value of this ... method 2: (1) and move some string concatenation out of inner loops. method 3: (2) and put the code inside a function -- accessing local variables is MUCH faster than global variables. Any script can do this. Many scripts should do this. method 4: (3) and accumulate strings in a list then join them and write them.See doucmentation for the basinhopping algorithm, which also works with multivariate scalar optimization. from scipy.optimize import basinhopping x0 = 0 sol ...Python programming has gained immense popularity in recent years due to its simplicity and versatility. Whether you are a beginner or an experienced developer, learning Python can ...scipy.optimize.OptimizeResult# class scipy.optimize. OptimizeResult [source] #. Represents the optimization result. Notes. Depending on the specific solver being used, OptimizeResult may not have all attributes listed here, and they may have additional attributes not listed here. Since this class is essentially a subclass of …Linear optimization problems with conditions requiring variables to be integers are called integer optimization problems. For the puzzle we are solving, thus, the correct model is: minimize y + z subject to: x + y + z = 32 2x + 4y + 8z = 80 x, y, z ≥ 0, integer. Below is a simple Python/SCIP program for solving it.Performance and optimization ... In this respect Python is an excellent language to work with, because solutions that look elegant and feel right usually are the best performing ones. As with most skills, learning what “looks right” takes practice, but one of …torch.optim. torch.optim is a package implementing various optimization algorithms. Most commonly used methods are already supported, and the interface is general enough, so that more sophisticated ones can also be easily integrated in the future.This paper presents a Python wrapper and extended functionality of the parallel topology optimization framework introduced by Aage et al. (Topology optimization using PETSc: an easy-to-use, fully parallel, open source topology optimization framework. Struct Multidiscip Optim 51(3):565–572, 2015). The Python interface, which simplifies …scipy.optimize.fsolve# scipy.optimize. fsolve (func, x0, args = (), fprime = None, full_output = 0, col_deriv = 0, xtol = 1.49012e-08, maxfev = 0, band = None, epsfcn = None, factor = 100, diag = None) [source] # Find the roots of a function. Return the roots of the (non-linear) equations defined by func(x) = 0 given a starting estimate ...When building for large scale use, optimization is a crucial aspect of software to consider. Optimized software is able to handle a large number of concurrent users or requests while maintaining the level of performance in terms of speed easily. This leads to overall customer satisfaction since usage is unaffected.Aug 25, 2022 · This leads to AVC denial records in the logs. 2. If the system administrator runs python -OO [APP] the .pyos will get created with no docstrings. Some programs require docstrings in order to function. On subsequent runs with python -O [APP] python will use the cached .pyos even though a different optimization level has been requested. Linear programming is a powerful tool for helping organisations make informed decisions quickly. It is a useful skill for Data Scientists, and with open-source libraries such as Pyomo it is easy to formulate models in Python. In this post, we created a simple optimisation model for efficiently scheduling surgery cases.SciPy optimize provides functions for minimizing (or maximizing) objective functions, possibly subject to constraints. It includes solvers for nonlinear …Sequential model-based optimization in Python. Getting Started What's New in 0.8.1 GitHub. Sequential model-based optimization. Built on NumPy, SciPy, and Scikit-Learn. Open source, …Towards Data Science. ·. 8 min read. ·. Jan 31, 2023. 4. Image by author. Table of contents. Introduction. Implementation. 2.1 Unconstrained …When building for large scale use, optimization is a crucial aspect of software to consider. Optimized software is able to handle a large number of concurrent users or requests while maintaining the level of performance in terms of speed easily. This leads to overall customer satisfaction since usage is unaffected.Feb 22, 2021 ... In this video, I'll show you the bare minimum code you need to solve optimization problems using the scipy.optimize.minimize method.Jan 31, 2021 · PuLP is a powerful library that helps Python users solve these types of problems with just a few lines of code. I have found that PuLP is the simplest library for solving these types of linear optimization problems. The objective function and constraints can all be added in an interesting layered approach with just one line of code each. CVXPY is a Python modeling framework for convex optimization ( paper ), by Steven Diamond and Stephen Boyd of Stanford (who wrote a textbook on convex optimization). In the way Pandas is a Python extension for dataframes, CVXPY is a Python extension for describing convex optimization problems.When building for large scale use, optimization is a crucial aspect of software to consider. Optimized software is able to handle a large number of concurrent users or requests while maintaining the level of performance in terms of speed easily. This leads to overall customer satisfaction since usage is unaffected.Linear programming is a powerful tool for helping organisations make informed decisions quickly. It is a useful skill for Data Scientists, and with open-source libraries such as Pyomo it is easy to formulate models in Python. In this post, we created a simple optimisation model for efficiently scheduling surgery cases.Python is one of the most popular programming languages in today’s digital age. Known for its simplicity and readability, Python is an excellent language for beginners who are just...Later, we will observe the robustness of the algorithm through a detailed analysis of a problem set and monitor the performance of optima by comparing the results with some of the inbuilt functions in python. Keywords — Constrained-Optimization, multi-variable optimization, single variable optimization.Sourcery is a static code analysis tool for Python. It uses advanced algorithms to detect and correct common issues in your code, such as typos, formatting errors, and incorrect variable names. Sourcery also offers automated refactoring tools that help you optimize your code for readability and performance.Visualization for Function Optimization in Python. By Jason Brownlee on October 12, 2021 in Optimization 5. Function optimization involves finding the input that results in the optimal value from an objective function. Optimization algorithms navigate the search space of input variables in order to locate the optima, and both the shape of the ...Latest releases: Complete Numpy Manual. [HTML+zip] Numpy Reference Guide. [PDF] Numpy User Guide. [PDF] F2Py Guide. SciPy Documentation.Are you a Python developer tired of the hassle of setting up and maintaining a local development environment? Look no further. In this article, we will explore the benefits of swit...If jac in [‘2-point’, ‘3-point’, ‘cs’] the relative step size to use for numerical approximation of jac. The absolute step size is computed as h = rel_step * sign (x) * max (1, abs (x)) , possibly adjusted to fit into the bounds. For method='3-point' the sign of h is ignored. If None (default) then step is selected automatically.7. Nlopt. This is a library for nonlinear local and global optimization, for functions with and without gradient information. It is designed as a simple, unified interface and packaging of several free/open-source nonlinear optimization libraries.Python is a powerful and versatile programming language that has gained immense popularity in recent years. Known for its simplicity and readability, Python has become a go-to choi...5 Python Optimization Methods 1. Python Profiling. Profiling is a way to programmatically analyze software bottlenecks. It involves analyzing memory usage, number of function calls, and the execution time of those calls. This analysis is important because it provides a way to detect slow or resource-inefficient parts of a software program ...In this article, some interesting optimization tips for Faster Python Code are discussed. These techniques help to produce result faster in a python code. Use builtin functions and libraries: Builtin functions like map () are implemented in C code. So the interpreter doesn’t have to execute the loop, this gives a …scipy.optimize.minimize — SciPy v1.12.0 Manual. scipy.optimize.minimize # scipy.optimize.minimize(fun, x0, args=(), method=None, jac=None, hess=None, …Aynı imkanı SciPy kütüphanesi Python dili için sağlıyor. SciPy bu fonksiyonu Nelder-Mead algoritması(1965) kullanarak gerçekliyor. ... The Nelder-Mead method is a heuristic optimization ...Feb 3, 2023 ... The selection of solver parameters or initial guesses can be determined by another optimization algorithm to search in among categorical or ...Description. Mathematical Optimization is getting more and more popular in most quantitative disciplines, such as engineering, management, economics, and operations research. Furthermore, Python is one of the most famous programming languages that is getting more attention nowadays. Therefore, we decided to …The capability of solving nonlinear least-squares problem with bounds, in an optimal way as mpfit does, has long been missing from Scipy. This much-requested functionality was finally introduced in Scipy 0.17, with the new function scipy.optimize.least_squares.. This new function can use a proper trust region algorithm …Visualization for Function Optimization in Python. By Jason Brownlee on October 12, 2021 in Optimization 5. Function optimization involves finding the input that results in the optimal value from an objective function. Optimization algorithms navigate the search space of input variables in order to locate the optima, and both the shape of the ...To better understand the Peephole optimization technique, let’s start with how the Python code is executed. Initially the code is written to a standard file, then you can run the command “python -m compileall <filename>”and get the same file in *.pyc format which is the result of the optimization. <Peephole> is a code …4 days ago ... Optimization (scipy.optimize) — SciPy v1.10.1 Manual Optimization ... Linear Programming and Optimization using Python Optimizing Python: Why ...Python is one of the most popular programming languages in today’s digital age. Known for its simplicity and readability, Python is an excellent language for beginners who are just...However, in contrast to compiled Code, I think the Python interpreter can not optimize bad style like explicit boolean comparisions, right? if condition == True: # do something. A compiler would optimize this and delete the == True part, but the interpreter always has to evaluate which statements wait after the condition == part, thus doing the ...Linear programming is a powerful tool for helping organisations make informed decisions quickly. It is a useful skill for Data Scientists, and with open-source libraries such as Pyomo it is easy to formulate models in Python. In this post, we created a simple optimisation model for efficiently scheduling surgery cases.Learn how to use scipy.optimize package for unconstrained and constrained minimization, least-squares, root finding, and linear programming. See examples of different optimization methods and options for multivariate scalar …. GEKKO is a Python package for machine learning and opti Chapter 9 : Numerical Optimization. 9.1. Finding th Python is a popular programming language used by developers across the globe. Whether you are a beginner or an experienced programmer, installing Python is often one of the first s...In this complete guide, you’ll learn how to use the Python Optuna library for hyperparameter optimization in machine learning.In this blog post, we’ll dive into the world of Optuna and explore its various features, from basic optimization techniques to advanced pruning strategies, feature selection, and … Performance and optimization ... In this respect Pyt Optimization Algorithm: We will use Scipy.optimize library from Python to implement the optimization. Let’s look at the code:-# Taking latest 6 weeks average of the base sales #-----# Ranking the date colume df_item_store_optimization ["rank"] = df_item_store_optimization["ds ... Tips and Tricks · Profile Your Code &mid...
Continue Reading